Imagine you’re building a house. You wouldn’t want to spend all your time crafting bricks – that’s why pre-made bricks exist. Django is like a pre-made brick set for back-end development in Python. It provides the essential building blocks you need to construct robust web applications, minus the hassle of starting from scratch. Django is a high-level Python web framework that encourages rapid development and clean, pragmatic design. It was created to help developers take applications from concept to completion as swiftly as possible.
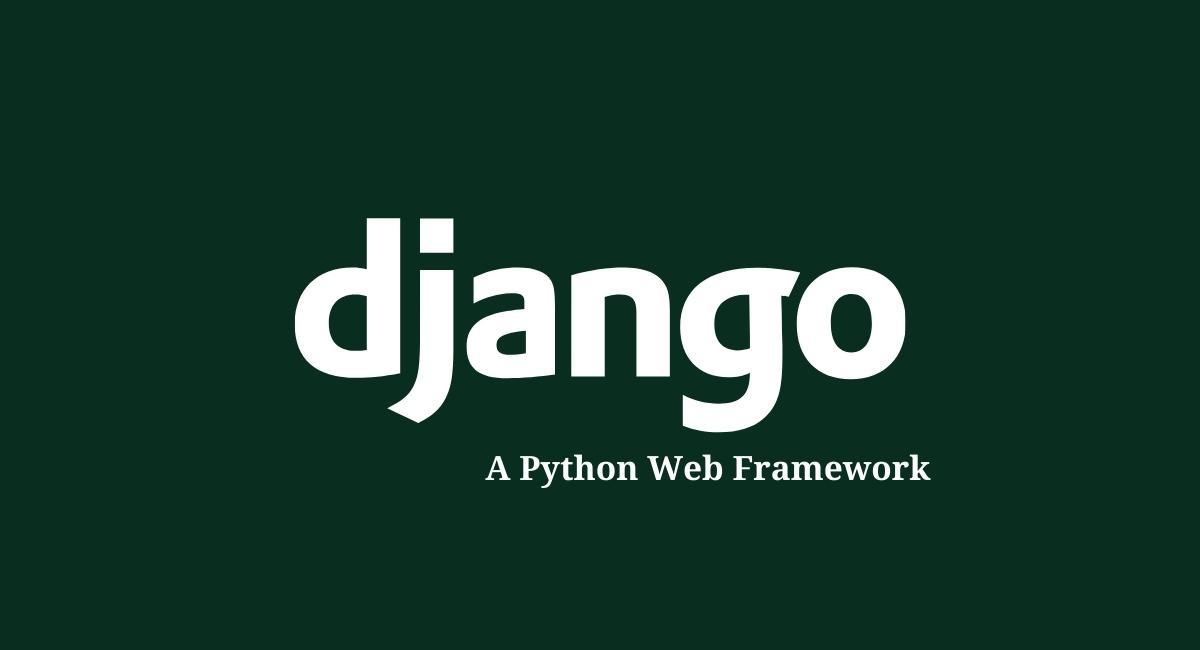
Key Features of Django:
Speed: Django follows the “batteries-included” philosophy. This means it comes packed with common back-end features like user logins, database connections, and API creation (APIs are the messengers between different parts of your web application). No need to reinvent the wheel – Django has it ready-made, saving you tons of development time.
Clean Code: Clean and organized code is key for any project. Django enforces a clear structure, making your back-end code easier to understand and maintain, for you and your fellow developers. Imagine a well-organized toolbox – that’s what Django does for your code.
Security Shield: Security is a top priority, especially when dealing with user data. Django prioritizes security and helps developers avoid common pitfalls that could leave their applications vulnerable. Think of it as a built-in security guard for your web application. Django includes robust security features out of the box, such as protection against SQL injection, cross-site scripting (XSS), cross-site request forgery (CSRF), and clickjacking.
Scalability: What if your website explodes in popularity? Django is built to handle that. It can effortlessly scale to accommodate a growing user base, so your back end won’t become a bottleneck for your success.
MVC (Model-View-Controller) Architecture:
- Model: Manages the data and database layer.
- View: Manages the user interface and presentation layer.
- Controller: Manages the business logic and user input handling.
- In Django, the term “MTV” (Model-Template-View) is often used instead, where “Template” refers to the presentation layer.
ORM (Object-Relational Mapping): Django includes a powerful ORM system that allows developers to interact with the database using Python code instead of raw SQL queries. This makes database operations easier and more secure.
URL Routing: Django’s URL dispatcher allows you to design clean and readable URL patterns for your application, making it easy to navigate and SEO-friendly.
Built-in Admin Interface: Django provides an automatically generated admin interface, which is a great tool for managing application data and users without additional coding.
Form Handling: Django simplifies form creation, validation, and processing, reducing the amount of boilerplate code needed for user input handling.
Extensible and Versatile: With its extensive ecosystem of third-party packages and plugins, Django can be tailored to suit a wide range of web development needs, from simple websites to complex web applications.
Comprehensive Documentation and Community Support: Django has excellent documentation and a vibrant community, which makes it easier for developers to find help, resources, and tutorials.
Whether you’re a back-end guru or just starting out, Django is a powerful tool to add to your arsenal. It simplifies development, promotes secure coding, and helps you build robust and scalable web applications with efficient APIs. So, if you want to up your back-end development game, Django is a champion worth considering.
What can you build with django?
With Django, you can build a wide variety of web applications, especially those that are complex and require a strong back-end. Here are some key areas where Django shines:
Complex Websites: Django is a great choice for websites that go beyond static content. Think news sites, e-commerce platforms, or social media applications. These websites typically involve user accounts, data management, and interactions between different parts of the application – all areas where Django’s features excel.
Content Management Systems (CMS): Django provides a solid foundation for building CMS systems. These are applications that allow users to easily create, edit, and publish content on a website, often without needing programming knowledge. Django’s ability to handle user roles, permissions, and content management makes it a popular choice for CMS development.
APIs (Application Programming Interfaces): APIs are the messengers between different parts of a web application, or even between different websites entirely. Django provides tools to build robust and secure APIs that allow data exchange and functionality sharing. This is crucial for modern web applications that often integrate with various services.
Data-Driven Applications: Django can be effectively used to build web applications that heavily rely on data processing and analysis. Its ability to connect to various databases and manipulate data efficiently makes it a valuable tool for developers working with data-centric projects.
Social Networking Platforms: The core functionalities Django offers, like user management, user interaction features, and secure communication, make it a suitable framework for developing social networking applications. Instagram: the massively popular photo and video sharing platform utilizes Django for its back-end functionality, handling user accounts, content management, and interactions between users.
Enterprise Applications: Django can be used to build complex and scalable web applications for businesses. Its ability to handle high traffic, manage user roles and permissions, and integrate with various services makes it a strong contender for enterprise-level development.
News Websites: The Washington Post: This prestigious newspaper leverages Django’s power for its web presence. Django manages user accounts, content delivery, and ensures a smooth user experience.
Video Streaming: Spotify, the popular music streaming service reportedly utilizes Django in its back-end infrastructure. It likely plays a role in user management, music recommendations, and playlist creation.
While these are some of the popular use cases, Django’s flexibility allows it to be adapted to a wider range of web development projects. If you’re looking for a framework that can handle complex back-end logic, user management, and API development, Django is worth considering.
Tools to get started with Django
1. Python: Django is a Python framework, so you’ll need Python installed on your system. You can download the latest version from https://www.python.org/downloads/
2. Text Editor or IDE: This is where you’ll write your Django code. A simple text editor like Notepad (Windows) or TextEdit (Mac) can work, but most developers prefer a more feature-rich option. Integrated Development Environments (IDEs) like PyCharm or Visual Studio Code offer functionalities like code completion, debugging tools, and project management, making development more efficient.
3. Virtual Environment (Optional but Recommended): A virtual environment helps isolate your project’s dependencies from your system-wide Python installation. This avoids conflicts and ensures you’re using the correct versions of libraries for your project. Tools like `venv` or `virtualenv` can be used to create virtual environments.
4. Code Editor Extensions (Optional): If you’re using a code editor like Visual Studio Code, there are extensions available specifically for Django development. These extensions can provide features like syntax highlighting, code snippets, and debugging assistance for Django code.
5. Database: Django applications typically store data in a relational database. Popular options include MySQL, PostgreSQL, and SQLite. You’ll need to have a database management system installed and configured to work with Django.
That’s the basic toolkit for getting started with Django development! There are additional tools you might encounter as you progress, but these will get you up and running.
How can I learn Django
There are several paths you can take to learn Django! Here are some options to consider:
1. Official Django Documentation:
The Django Project itself has excellent documentation that covers everything from installation and setup to advanced topics. It’s a comprehensive resource and a great place to start: [https://docs.djangoproject.com/en/5.0/]
2. Tutorials:
Many websites offer Django tutorials, ranging from beginner-friendly to more in-depth explorations. These tutorials often walk you through building a small application, which is a great way to learn by doing. Here are a couple of popular options:
Django Girls Tutorial: [https://djangogirls.org/](https://djangogirls.org/]
Django Tutorial on W3Schools:[https://www.w3schools.com/django/]
3. Online Courses:
Several online platforms offer Django courses, both free and paid. These courses can provide structured learning paths with video lectures, quizzes, and assignments. Here are some popular platforms to explore:
Coursera
Udemy
LinkedIn Learning
4. Books:
There are many books available on Django development. These can offer a deeper dive into the framework and best practices. A couple of highly rated options include:
“Two Scoops of Django” by Daniel Greenfeld and Audrey Roy Greenfeld
“Django for Professionals” by William Vincent
5. Video Tutorials:
YouTube offers a wealth of video tutorials on Django development. These can be a good option for visual learners who prefer a more hands-on approach.
Learning Tips
Start with the Basics: Before diving into Django, ensure you have a solid understanding of Python and basic web development concepts like HTML, CSS, and SQL.
Practice with Small Projects: Once you grasp the fundamentals, try building small Django applications to solidify your learning. There are many project ideas available online.
Join the Django Community: The Django community is large and active. There are online forums, meetups, and Stack Overflow threads where you can ask questions and connect with other Django developers.
Learning Django takes time and dedication, but with the right resources and a commitment to practice, you can become proficient in this powerful web development framework.
Django is a powerful tool that can unlock a world of web development possibilities. Whether you’re a seasoned programmer or just starting your coding journey, Django offers a streamlined approach to building robust and secure back-end applications.
With its clear structure, extensive features, and supportive community, Django can empower you to turn your web app ideas into reality. So, if you’re ready to dive in and experience the power of Django, there’s no better time to start than now!
Thank you for reading this article. You can learn more on our blog.